I made an Oopsie or: How to request a new Telegram BotToken
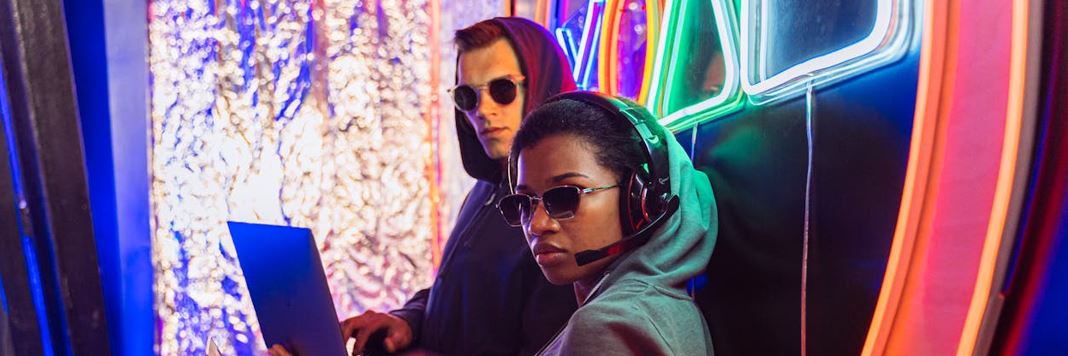
Always keep track to which repository you are committing...
Only showing article with this tag: Telegram
Always keep track to which repository you are committing...
Now that I got comments, I want to be notified if I have new comments waiting for approval.
Get monitoring alerts to your mobile using Icinga2 and a Telegram Bot